public
Authored by
bzctoons 
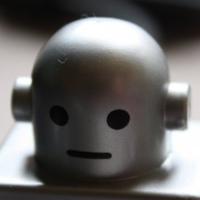

split a string as list with double quotes (") handling
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
#include <QDebug>
#include <QUrl>
/**
* @brief stringToList
* @abstract split a string as list with double quotes (") handling
* @param line
* @return QStringList
*/
QStringList stringToList(QString line) {
QStringList list;
QRegExp rx("\"([^\"]*)\"");
int pos = 0;
while ((pos = rx.indexIn(line, pos)) != -1) {
list << rx.cap(0);
pos += rx.matchedLength();
}
for (auto l : qAsConst(list)) {
QString ol = l;
l.replace(" ", "<SPC>");
line.replace(ol, l);
}
list.clear();
QStringList tmp = line.split(' ');
for (auto l : qAsConst(tmp)) {
if (l.isEmpty())
continue;
list << l.replace("<SPC>", " ");
}
return list;
}
int main(int argc, char *argv[]) {
QStringList l;
l << "helo"
<< "réda"
<< "multi word"
<< "\\plok\\"
<< "\"plok is my name\\"
<< "SEARCH \"testme please\" LAST_MODIFIED:2 days CREATED:31/12/2020 MAX_SIZE:1M EXT:txt,doc,xlsx TYPE:image OR "
"text"
;
for (const auto &i : qAsConst(l)) {
qDebug() << stringToList(i);
qDebug() << QUrl::toPercentEncoding(i);
}
return 0;
}
Please register or sign in to comment